Creative Coding 개발환경 설정 및 기본 프로젝트
글 작성자: 택시 운전사
반응형
개발 환경 설정
코드 에디터
Visual Studio Code 확장 프로그램
- ESlint, jslint - JavaScript용 코드 lint 확장 프로그램
- Beautify - 코드 포메터 확장 프로그램
- Live Server - HTML 파일을 서빙하는 가상의 서버를 띄우는 확장 프로그램
추가 팁
- 파일이 크지 않기 때문에 로컬영역이 아닌 클라우드에 저장해서 사용하는 것도 좋다.
- 구글 드라이브 + 드라이브 파일 스트림으로 로컬 드라이브처럼 사용 가능)
기본 프로젝트
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>test</title> <link rel="stylesheet" href="style.css"> </head> <body> <script type="module" src="app.js"></script> </body> </html>
style.css
* { outline: 0; margin: 0; padding: 0; } html { width: 100%; height: 100%; } body { width: 100%; height: 100%; background-color: #b34ec7; } canvas { width: 100%; height: 100%; }
app.js
- requestAnimationFrame - 브라우저에게 수행하기를 원하는 애니메이션을 알리고 다음 리페인트가 진행되기 전에 해당 애니메이션을 업데이트하는 함수를 호출하게 하는 함수, 재생 시간대마다 달라지는 음악의 파형을 표현하기 위해 사용하기도 한다.
- devicePixelRatio - 현재 표시 장치의 물리적 픽셀과 CSS 픽셀의 비율
- 캔버스를 이용한 도형 그리기
class App { constructor() { this.canvas = document.createElement('canvas'); document.body.appendChild(this.canvas); this.ctx = this.canvas.getContext('2d'); // 2D 렌더링 컨텍스트 생성 this.pixelRatio = window.devicePixelRatio > 1 ? 2 : 1; window.addEventListener('resize', this.resize.bind(this), false); this.resize() window.requestAnimationFrame(this.animate.bind(this)); } resize() { this.stageWidth = document.body.clientWidth; this.stageHeight = document.body.clientHeight; this.canvas.width = this.stageWidth * this.pixelRatio; this.canvas.height = this.stageHeight * this.pixelRatio; this.ctx.scale(this.pixelRatio, this.pixelRatio) } animate() { window.requestAnimationFrame(this.animate.bind(this)); this.ctx.clearRect(0,0, this.stageWidth, this.stageHeight); // canvas의 사각형 영역 지우기 this.ctx.fillStyle = '#f0d785'; this.ctx.beginPath(); // 도형 그리기 시작 this.ctx.arc( this.stageWidth /2, // 중심축의 x좌표 this.stageHeight / 2, // 중심축의 y좌표 300, // 반지름 0, // 시작 각도 2* Math.PI, // 종료 각도 true // true일 시 반시계방향, false일 시 시계방향, 값을 안 넣었을 때에는 시계방향이 기본값 ) this.ctx.fill(); } } window.onload = () => { new App(); // 새로운 앱 인스턴스 생성 }
결과물
원 하나를 캔버스에 그린다.
기존 코드에서 색상값 정도만 바꾸었다.
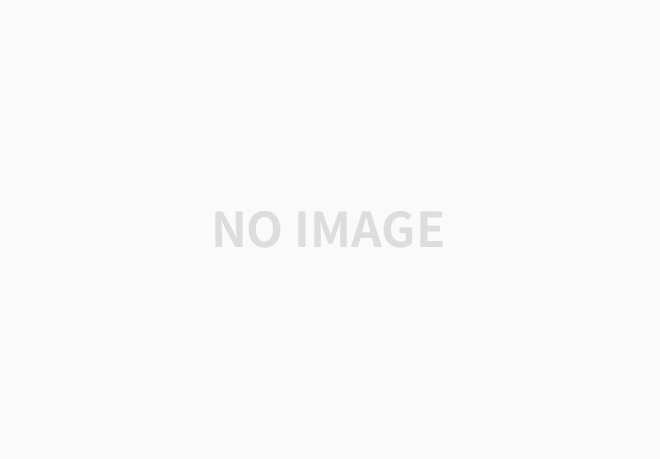
반응형
'Web > JavaScript' 카테고리의 다른 글
HTML5 Canvas Tutorial : 화면에 튕기는 공 만들기 (0) | 2020.12.06 |
---|---|
자바스크립트로 HTML5 캔버스에 회전하는 도형 만들기 (0) | 2020.11.28 |
Creative Coding을 들어가며 (0) | 2020.11.14 |
[JavaScript] {} + {}의 결과는 뭘까? (0) | 2019.12.14 |
[JavaScript] Ajax (0) | 2019.12.11 |
댓글
이 글 공유하기
다른 글
-
HTML5 Canvas Tutorial : 화면에 튕기는 공 만들기
HTML5 Canvas Tutorial : 화면에 튕기는 공 만들기
2020.12.06 -
자바스크립트로 HTML5 캔버스에 회전하는 도형 만들기
자바스크립트로 HTML5 캔버스에 회전하는 도형 만들기
2020.11.28 -
Creative Coding을 들어가며
Creative Coding을 들어가며
2020.11.14 -
[JavaScript] {} + {}의 결과는 뭘까?
[JavaScript] {} + {}의 결과는 뭘까?
2019.12.14
댓글을 사용할 수 없습니다.